Bootstrap Layout
Let’s learn about what is Bootstrap Layout with Container Row and Columns in bootstrap. Bootstrap’s layout system is based on a grid structure that ensures responsive and flexible designs. It uses containers, rows, and columns to organize and align content in a way that adapts to different screen sizes.
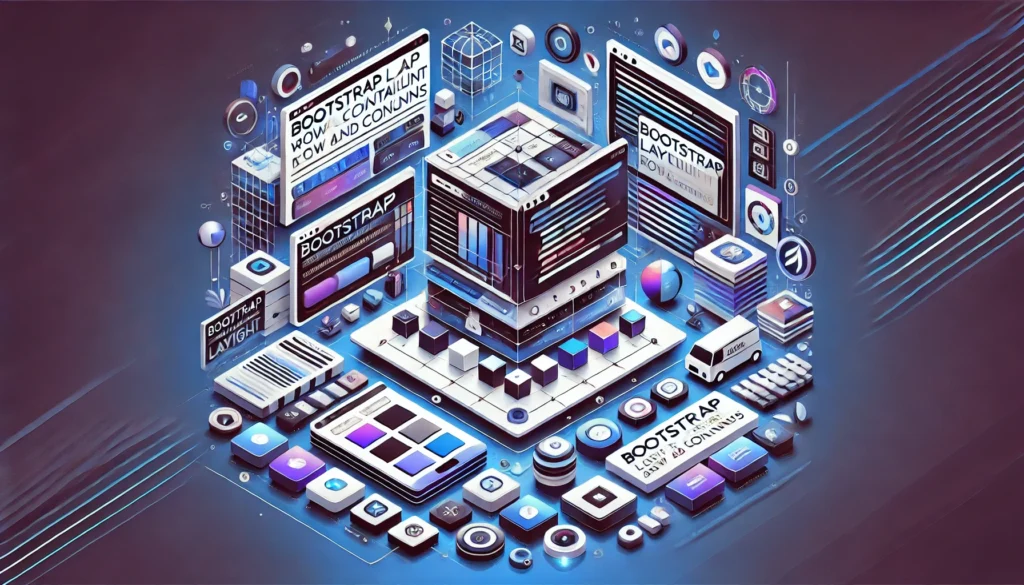
1. Container
The container is the outermost wrapper that holds rows and columns. It provides the necessary padding and centers the content on the page.
- Types of Containers:
.container
: Provides a fixed-width container with predefined breakpoints..container-fluid
: Spans the entire width of the viewport..container-{breakpoint}
: Applies a fixed-width container only at a specific breakpoint (sm
,md
,lg
, etc.).
Example:
<div class="container">
<!-- Content here -->
</div>
2. Row
A row is used to create a horizontal group of columns. It ensures proper alignment and spacing between the columns.
- Key Points:
- Rows are placed inside containers.
- Use
.row
class to create a row. - Rows use a negative margin to offset the padding of the container.
Example:
<div class="container">
<div class="row">
<!-- Columns will go here -->
</div>
</div>
3. Columns
Columns are the building blocks of the layout and are nested within rows. Bootstrap’s grid system divides the layout into 12 equal columns.
- Key Features:
- Use
.col-{breakpoint}-{number}
to define the column size and how much space it should take. - Columns are responsive and can adjust for different screen sizes using breakpoints (
sm
,md
,lg
,xl
,xxl
). - Unspecified columns share equal space.
- Use
Example:
<div class="container">
<div class="row">
<div class="col-md-4">Column 1</div>
<div class="col-md-4">Column 2</div>
<div class="col-md-4">Column 3</div>
</div>
</div>
Full Example: Combining Container, Row, and Columns
Here’s a complete layout example:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Bootstrap Layout Example</title>
<link href="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0/dist/css/bootstrap.min.css" rel="stylesheet">
</head>
<body>
<!-- Container -->
<div class="container">
<!-- Row -->
<div class="row">
<!-- Column 1 -->
<div class="col-md-6 col-lg-4 bg-primary text-white text-center">
Column 1
</div>
<!-- Column 2 -->
<div class="col-md-6 col-lg-4 bg-secondary text-white text-center">
Column 2
</div>
<!-- Column 3 -->
<div class="col-md-12 col-lg-4 bg-success text-white text-center">
Column 3
</div>
</div>
</div>
<script src="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0/dist/js/bootstrap.bundle.min.js"></script>
</body>
</html>
Explanation:
- Container: Wraps the entire content and centers it.
- Row: Groups the columns horizontally.
- Columns:
col-md-6
: Takes 6 out of 12 columns on medium screens.col-lg-4
: Takes 4 out of 12 columns on large screens.
Result:
- On small screens: Columns stack vertically.
- On medium screens: Two columns side by side.
- On large screens: Three columns side by side.
So, that is all about Bootstrap Layout with Container Row and Columns. You can also learn What is Bootstrap? How to use bootstrap in your webpage?